官方文档:https://gorm.io/zh_CN/docs/connecting_to_the_database.html
最简单的连接方法
通过一行 DSN
连接
1 2
| dsn := "user:pass@tcp(127.0.0.1:3306)/dbname?charset=utf8mb4&parseTime=True&loc=Local" db, err := gorm.Open(mysql.Open(dsn), &gorm.Config{})
|
示例
1 2 3 4 5 6 7 8 9 10 11 12 13
| package main
import ( "fmt" "gorm.io/driver/mysql" "gorm.io/gorm" )
func main() { dsn := "root:1234@tcp(127.0.0.1:3306)/gorm_learning?charset=utf8mb4&parseTime=True&loc=Local" db, err := gorm.Open(mysql.Open(dsn), &gorm.Config{}) fmt.Println(db, err) }
|
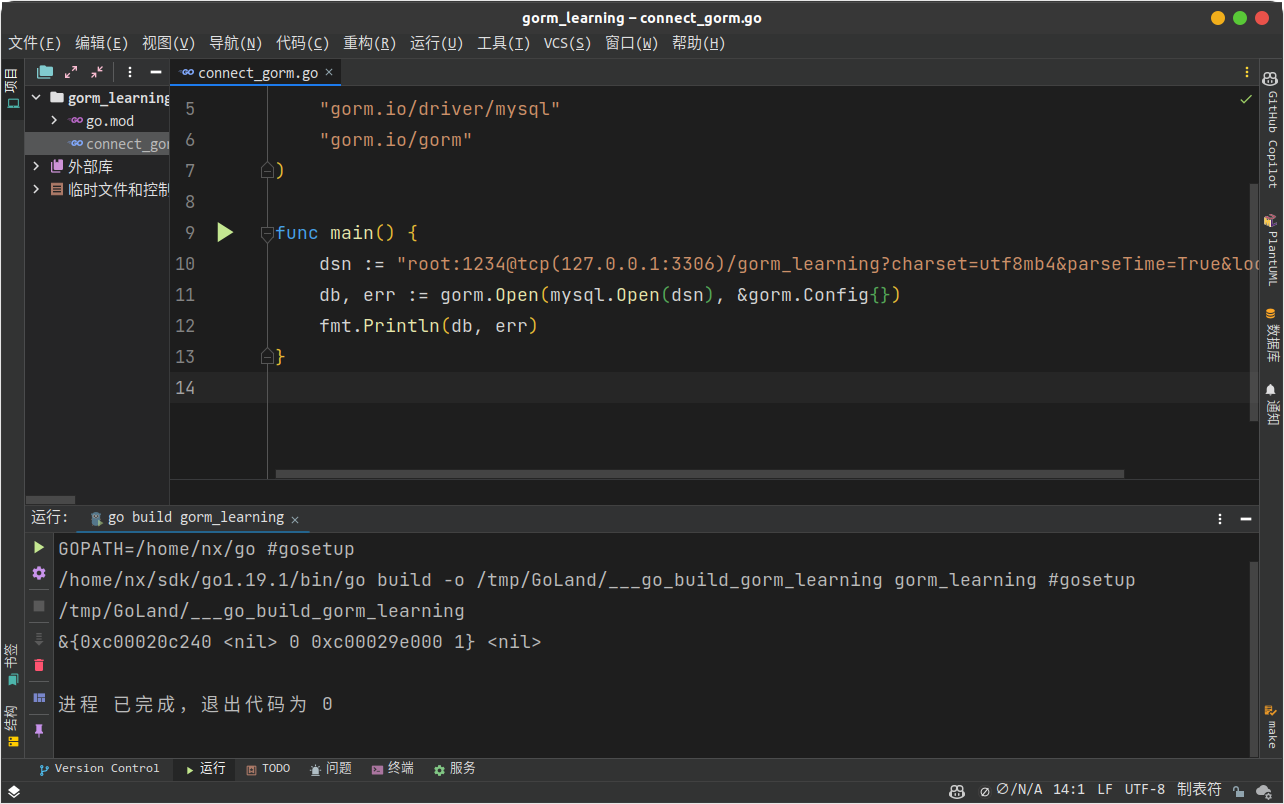
可见没有返回错误
配置连接参数
gorm.Open()
方法有两个参数,分别可以对数据库和 GORM 进行更高级的配置
mysql.Config
这样可以设置一些高级配置
1 2 3 4 5 6 7 8
| db, err := gorm.Open(mysql.New(mysql.Config{ DSN: "gorm:gorm@tcp(127.0.0.1:3306)/gorm?charset=utf8&parseTime=True&loc=Local", DefaultStringSize: 256, DisableDatetimePrecision: true, DontSupportRenameIndex: true, DontSupportRenameColumn: true, SkipInitializeWithVersion: false, }), &gorm.Config{})
|
更多的可以配置的项目可以点进去看源码,或者去看文档
gorm.Config
这个自己去看官方文档吧,感觉还是挺详细的
1 2 3 4 5 6 7 8 9 10 11 12
| type Config struct { SkipDefaultTransaction bool NamingStrategy schema.Namer Logger logger.Interface NowFunc func() time.Time DryRun bool PrepareStmt bool DisableNestedTransaction bool AllowGlobalUpdate bool DisableAutomaticPing bool DisableForeignKeyConstraintWhenMigrating bool }
|
比较重点的有命名策略,这个可以更改你创建的表名,增加些前缀后缀什么的
然后还有最后一个 DisableForeignKeyConstraintWhenMigrating
是否要跳过外键约束,这个建议跳过
因为这个交给数据库检查的话代价很高,建议自己在代码逻辑自动体现外键关系(逻辑外键)
示例:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27
| package main
import ( "fmt" "gorm.io/driver/mysql" "gorm.io/gorm" "gorm.io/gorm/schema" )
func main() { db, err := gorm.Open(mysql.New(mysql.Config{ DSN: "root:1234@tcp(127.0.0.1:3306)/gorm_learning?charset=utf8mb4&parseTime=True&loc=Local", DefaultStringSize: 191, DisableDatetimePrecision: true, DontSupportRenameIndex: true, DontSupportRenameColumn: true, SkipInitializeWithVersion: false, }),前 *gorm.DB 返回一个通 &gorm.Config{ NamingStrategy: schema.NamingStrategy{ TablePrefix: "t_", SingularTable: true, }, DisableForeignKeyConstraintWhenMigrating: true, }) fmt.Println(db, err) }
|
使用连接池
与数据库连接时可以使用连接池,关于连接池可以看这篇 Golang 侧数据库连接池原理和参数调优
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| sqlDB, err := db.DB()
sqlDB.SetMaxIdleConns(10)
sqlDB.SetMaxOpenConns(100)
sqlDB.SetConnMaxLifetime(time.Hour)
func GetDB() *gorm.DB { return db }
|