ORM 是把结构和数据库表创建一个映射,所以我们先建一个结构体(也就是模型,这个后面详细讲)
1 2 3 4
| type User struct { Name string }
|
使用 AutoMigrate 自动迁移/建表
尝试使用 db.AutoMigrate()
方法自动创建表
1
| err = db.AutoMigrate(&User{})
|
再次观察,发现多了个叫 t_user
的表
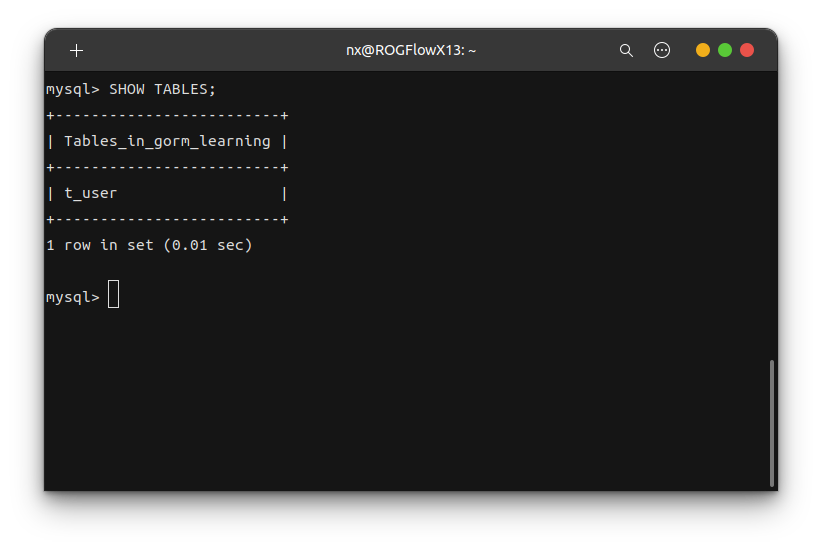
为什么会多前缀呢?这是在创建连接的时候,在 gorm.Config
中预设的
类似地还可以有单复数后缀
1 2 3 4
| NamingStrategy: schema.NamingStrategy{ TablePrefix: "t_", SingularTable: true, }
|
将其设置为 false
再次执行,新建表的命名格式是复数
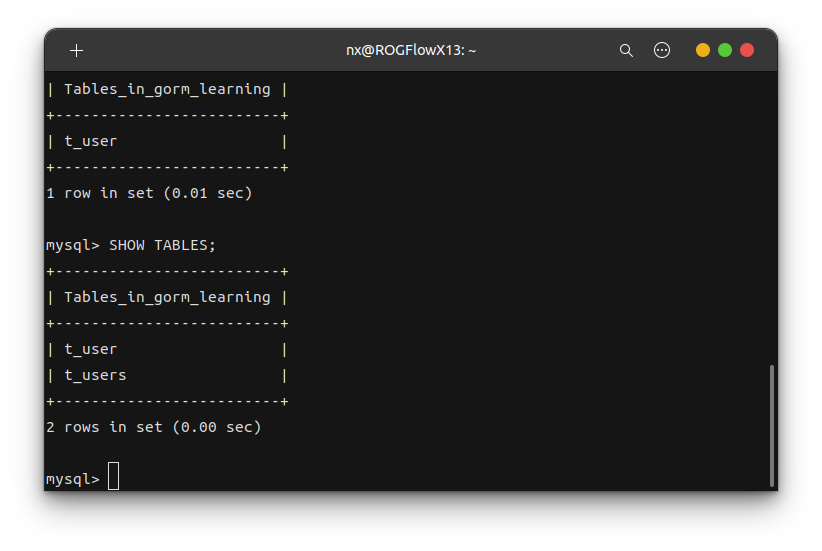
使用 Migrator 接口
GORM 提供了 Migrator 接口,我们可以直接使用这个接口来操作
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35
| type Migrator interface { AutoMigrate(dst ...interface{}) error
CurrentDatabase() string FullDataTypeOf(*schema.Field) clause.Expr
CreateTable(dst ...interface{}) error DropTable(dst ...interface{}) error HasTable(dst interface{}) bool RenameTable(oldName, newName interface{}) error GetTables() (tableList []string, err error)
AddColumn(dst interface{}, field string) error DropColumn(dst interface{}, field string) error AlterColumn(dst interface{}, field string) error MigrateColumn(dst interface{}, field *schema.Field, columnType ColumnType) error HasColumn(dst interface{}, field string) bool RenameColumn(dst interface{}, oldName, field string) error ColumnTypes(dst interface{}) ([]ColumnType, error)
CreateConstraint(dst interface{}, name string) error DropConstraint(dst interface{}, name string) error HasConstraint(dst interface{}, name string) bool
CreateIndex(dst interface{}, name string) error DropIndex(dst interface{}, name string) error HasIndex(dst interface{}, name string) bool RenameIndex(dst interface{}, oldName, newName string) error }
|
CreateTable()
创建表
尝试一下代码,可以起到一样的作用
1 2
| M := db.Migrator() err = M.CreateTable(&User{})
|
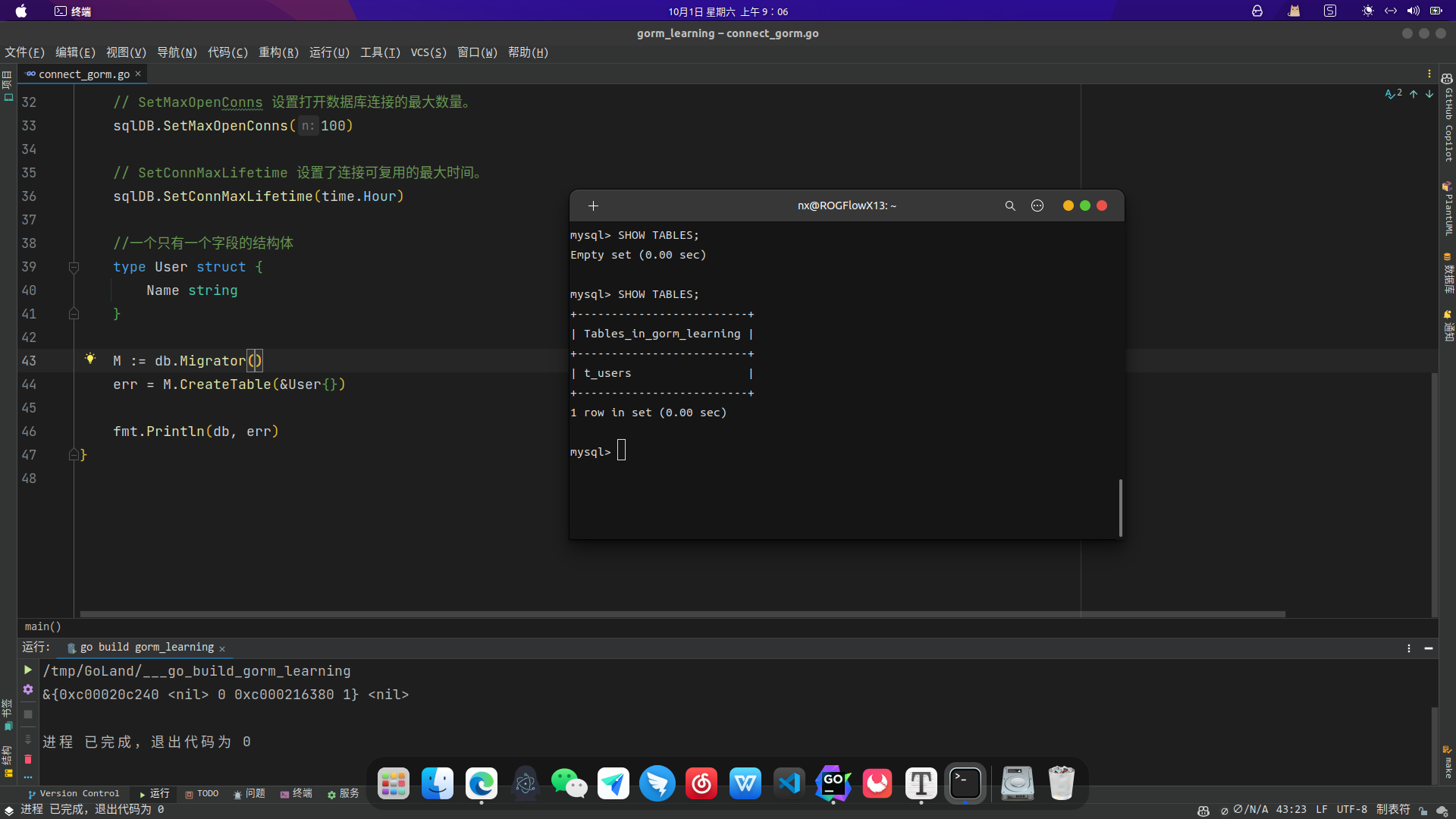
HasTable()
是否存在表
调用 HasTable()
方法即可
1 2
| M := db.Migrator() fmt.Println(M.HasTable(&User{}))
|
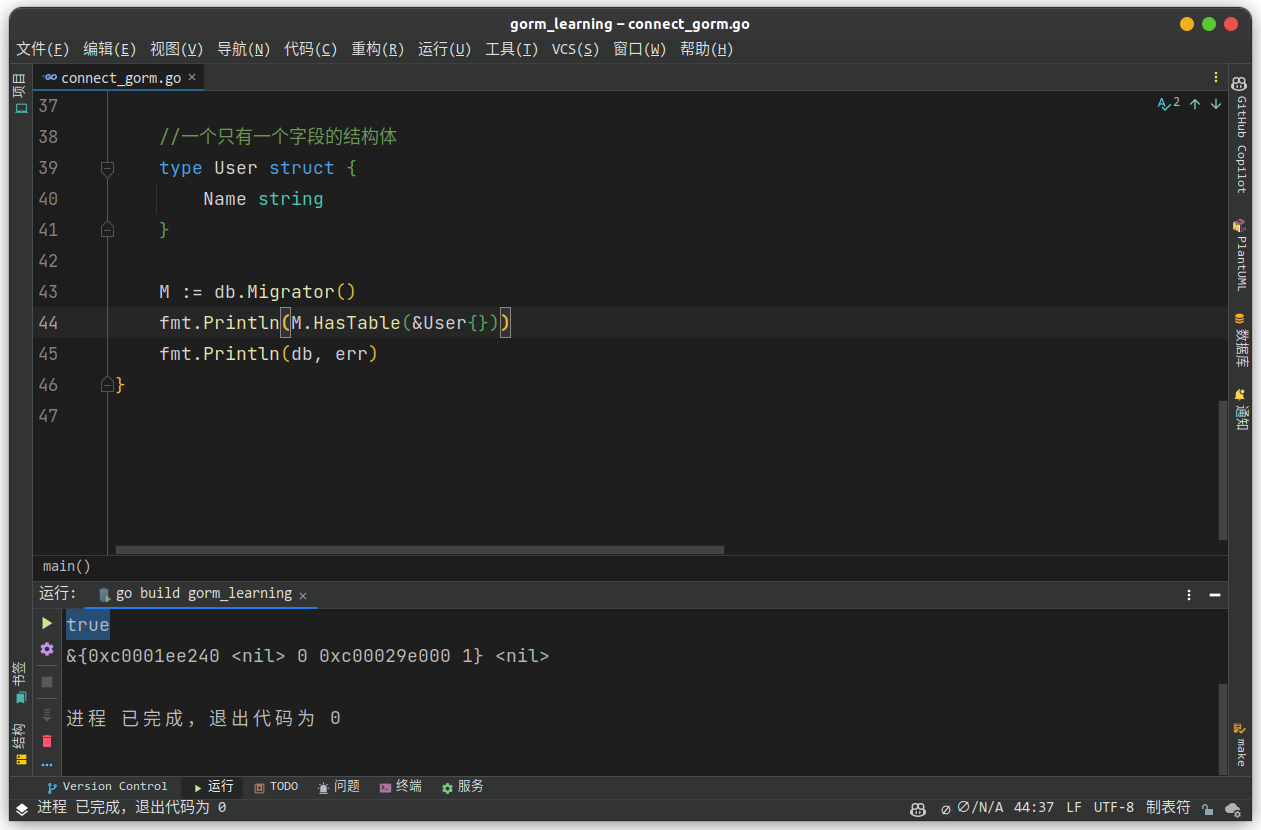
这里还可以以表名的形式去查
1 2
| M := db.Migrator() fmt.Println(M.HasTable("t_users"))
|
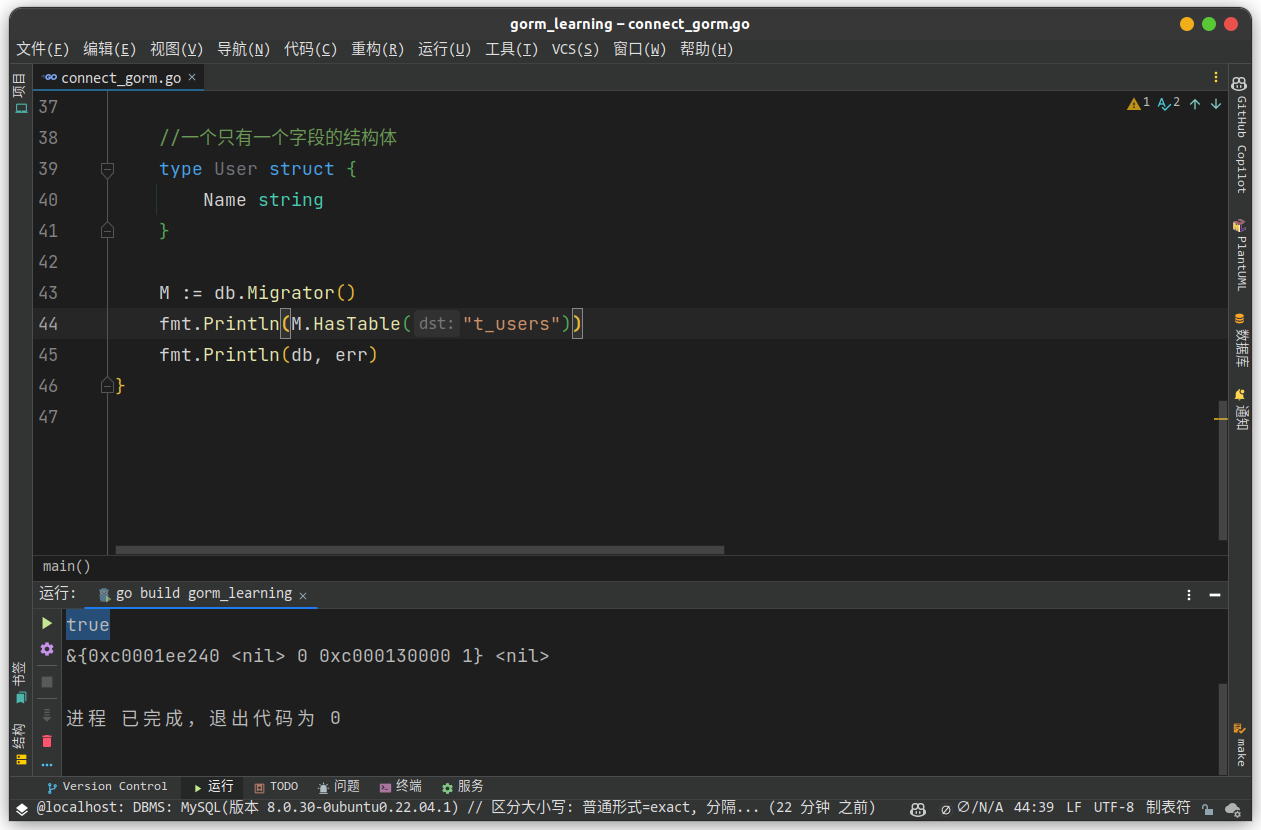
DropTable()
删除表
这个也很简单易懂,并且同时支持结构体和表名的表示法
1 2 3 4
| M := db.Migrator() fmt.Println(M.DropTable(&User{}))
fmt.Println(M.DropTable("t_users"))
|
1 2
| db.Migrator().RenameTable(&User{}, &UserInfo{}) db.Migrator().RenameTable("users", "user_infos")
|
但是你使用表名重命名了之后就无法操纵这个表了,所以还是建议使用结构体的方式
其他方法可以去看文档,关于列的,关于约束,的关于索引的